How to understand COBOL copybook?
You use the COPY statement to include a copybook in a program. This statement is used in the Working-Storage Section of your COBOL program. The COPY statement tells the compiler to insert the contents of the specified copybook into your program at the point where the statement appears.
Let’s break down how copybooks work:
Data Structures: Copybooks primarily define data structures, which are essentially blueprints for how data is organized and stored. They define the data types, names, and sizes of the different fields that make up your data. For example, you could have a copybook that defines a customer record structure, with fields for customer name, address, phone number, etc.
Reusability: The real beauty of copybooks is their reusability. You can define a copybook once and then use it in multiple programs. This avoids code duplication and ensures consistency across your applications. For example, if you have a copybook that defines a customer record structure, you can use that same copybook in your order processing programs, customer service programs, and any other program that needs to work with customer data.
Maintainability: Copybooks make your code easier to maintain. If you need to change a data structure, you only need to make the change in the copybook. This change will automatically be reflected in all the programs that use the copybook.
Let’s look at a simple example:
“`cobol
IDENTIFICATION DIVISION.
PROGRAM-ID. MY-PROGRAM.
DATA DIVISION.
WORKING-STORAGE SECTION.
COPY CUSTOMER-RECORD.
PROCEDURE DIVISION.
…
“`
In this example, the COPY CUSTOMER-RECORD statement includes the contents of the CUSTOMER-RECORD copybook in the Working-Storage Section of your program. This copybook would contain the definition of the customer record structure, as mentioned earlier.
By understanding copybooks, you can write more efficient, maintainable, and organized COBOL programs.
What is the .cpy file COBOL?
In essence, a copybook file in COBOL is a powerful tool used to define data elements that can be reused across multiple programs. Imagine it as a blueprint for your data structure. When you want to use the same data structure in different parts of your COBOL code, instead of rewriting the entire structure each time, you can simply reference the copybook file.
Think of it like this: Imagine you are building a house. You might have a standard blueprint for doors, windows, and walls. You can use this blueprint repeatedly for every room in your house. In COBOL, your copybook file is like this blueprint, holding the definitions for data elements that can be used in various programs.
Here’s how it works: The Declaration Generator, a part of the COBOL compiler, creates a copybook file (.cpy) that holds the definitions for your data elements. When you use the COPY statement in your COBOL program, the compiler inserts the contents of the copybook file into your program. This allows you to avoid repetitive code and ensure consistency across your application.
Let’s explore a simple example. Suppose you have a program that needs to store information about customers, including their name, address, and phone number. Instead of defining these data elements in every program that needs them, you can create a copybook file (CUSTOMER.cpy) containing these definitions.
In the CUSTOMER.cpy file, you would have:
“`cobol
01 CUSTOMER-RECORD.
05 CUSTOMER-NAME PIC X(30).
05 CUSTOMER-ADDRESS PIC X(50).
05 CUSTOMER-PHONE PIC X(10).
“`
Now, in any program that needs to work with customer data, you simply use the COPY statement like this:
“`cobol
COPY CUSTOMER.
“`
This will insert the definitions from the CUSTOMER.cpy file into your program, making the CUSTOMER-RECORD available for use.
The advantage of this approach is clear: You maintain your code’s readability and consistency by avoiding duplicate code. If you need to change the customer record structure, you only need to make the change in the copybook file; your programs will automatically use the updated definitions.
This simple concept of copybooks in COBOL is a powerful mechanism that promotes code reuse, efficiency, and maintainability.
What is the use of copybook in mainframe?
Let’s break down how copybooks work their magic:
Source Definition: Imagine you have a file with customer data. The source definition tells us the basic layout of that data – things like the length of each field (name, address, phone number), the type of data (text, numbers), and their order.
Copybook’s Role: The copybook takes this source definition and adds extra information you need to work with the data effectively. It’s like adding annotations to your blueprint, detailing how you want to manipulate the data. This could include things like:
Formatting: How do you want the data to be displayed?
Reformatting: Do you need to change the data format to work with another system?
Record Selection: Do you only want to work with specific customers?
Data Creation: Do you need to create new data based on the existing information?
The Power of Logical View:
The copybook, by combining the source definition and your additional information, creates a logical view of the data. This logical view is essential for programs that work with the data. It lets programs understand the data’s structure, perform calculations, and access specific pieces of information. Think of it like a map that allows programs to navigate and work with the data efficiently.
Think of it this way:
The copybook is like a translator, bridging the gap between the raw data in the file and the programs that need to use it. It makes the data understandable and accessible, ensuring that programs can work with it effectively. Without the copybook, working with mainframe data would be like trying to build a house without a blueprint – chaotic and inefficient!
What is the difference between include and copybook in COBOL?
INCLUDE is a precompiler directive that’s used to insert the contents of a file into the main program during the compilation process. Think of it like a “cut and paste” operation that happens before the COBOL compiler actually gets to work. This means that the included file’s content is merged with your main program’s code, and then the combined code is compiled as one unit. This makes it easier to maintain and reuse code snippets across multiple programs.
COPYBOOK, on the other hand, is a COBOL verb that acts as a placeholder for a separate file. The actual content of the file is copied in during the compilation process. The compiler sees this verb and replaces it with the contents of the file it references. This allows you to define data structures, tables, and other common elements in a separate file and reuse them across different COBOL programs without needing to rewrite them every time.
Now, let’s clarify the subtle difference between how INCLUDE and COPYBOOK handle the expansion of their referenced files. INCLUDE, being a precompiler directive, expands the referenced file’s content right away when it retrieves the program from the source repository. This means you’ll have a combined program file that includes all the INCLUDEd content before the compiler even starts working.
COPYBOOK works a bit differently. The COPYBOOK verb acts as a placeholder in your main program. It is only during the compilation process that the COBOL compiler actually goes and retrieves the contents of the referenced file and replaces the COPYBOOK verb with that content. This means that the COPYBOOK expansion happens later in the compilation process, as opposed to the pre-compilation stage used by INCLUDE. While both achieve the same goal of integrating reusable code into your programs, the timing and approach are slightly different.
So, INCLUDE is all about pre-compilation, while COPYBOOK focuses on the actual compilation process. Understanding these differences helps you choose the right tool for your coding needs.
How do I read a tape file in mainframe?
Here’s how it works:
1. The IEBGENER Utility: This powerful utility is like a file converter for mainframes. It lets you move data between different file types, including tape files and sequential files.
2. The Process: You’ll use JCL (Job Control Language) to tell the IEBGENER utility what to do. The JCL will specify the tape file you want to copy, the sequential file where you want the data to go, and any other relevant details.
3. The Sequential File: Once IEBGENER completes the copy, you’ll have a sequential file containing the data from your tape. You can then easily view or process this file using standard mainframe tools like ISPF or TSO.
Let’s take a look at a sample JCL that you can use to copy a tape file to a sequential file using IEBGENER:
“`
//COPYTP JOB (YOURID),CLASS=A
//STEP1 EXEC PGM=IEBGENER
//SYSPRINT DD SYSOUT=A
//SYSIN DD DSN=YOUR.JCL(COPYTP),DISP=SHR
//SYSUT1 DD DSN=YOUR.TAPE,DISP=(OLD,KEEP),UNIT=TAPE,VOL=SER=VOLUMESER
//SYSUT2 DD DSN=YOUR.SEQUENTIAL,DISP=(NEW,KEEP),SPACE=(CYL,(1,1))
“`
Explanation:
//COPYTP JOB: This line defines the job name and some basic parameters.
//STEP1 EXEC PGM=IEBGENER: This line tells the system to execute the IEBGENER program.
//SYSPRINT DD SYSOUT=A: This line specifies that the output should be directed to the system output (SYSOUT).
//SYSIN DD DSN=YOUR.JCL(COPYTP),DISP=SHR: This line identifies the JCL file that contains the instructions for IEBGENER.
//SYSUT1 DD DSN=YOUR.TAPE,DISP=(OLD,KEEP),UNIT=TAPE,VOL=SER=VOLUMESER: This line specifies the tape file you want to copy. Replace “YOUR.TAPE” with the actual name of your tape file, and “VOLUMESER” with the volume serial number of the tape.
//SYSUT2 DD DSN=YOUR.SEQUENTIAL,DISP=(NEW,KEEP),SPACE=(CYL,(1,1)): This line defines the sequential file where the data will be stored. Replace “YOUR.SEQUENTIAL” with the desired name for your sequential file.
Important Notes:
Replace the placeholders: Make sure to replace “YOURID”, “YOUR.JCL”, “YOUR.TAPE”, “VOLUMESER”, and “YOUR.SEQUENTIAL” with your actual values.
Tape Volume: You’ll need to know the volume serial number of the tape to access it.
JCL: This JCL example is a basic starting point. You might need to adjust it based on the specific requirements of your tape file and the environment where you’re running the job.
Error Handling: It’s a good idea to add error handling to your JCL to capture any problems that might occur during the copy process.
Remember, this process is essential for working with tape files on mainframe systems. Once you’ve copied the tape data to a sequential file, you can use a variety of tools to read, browse, and process the information.
How to find the length of a copybook?
Let’s break down how this works.
Compiling with the Copybook: When you compile a program, it reads the copybook. This tells the compiler how to define and handle the data structures your program will work with.
The Data Map: The compiler creates a data map to represent this information. This map usually includes the size of each data field defined in the copybook.
Finding the Length: By looking at the data map, you can add up the sizes of all the data fields defined in your copybook. This total sum represents the length of the data structure.
Example:
Let’s say you have a copybook with two fields:
Field A: A 10-byte character field.
Field B: A 5-byte numeric field.
After compiling, you will see the data map, which shows the size of each field. Field A is 10 bytes long, and Field B is 5 bytes long. The total length of your data structure would be 15 bytes (10 bytes + 5 bytes).
Important Note: The exact format and location of the data map can vary depending on the compiler and programming language you are using. Consult your compiler documentation for the specifics.
Beyond the Basics:
Sometimes, it’s not as simple as just adding up the field sizes. Here are a few things to consider:
Padding: Compilers often add extra padding (empty space) between fields to align the data in memory efficiently. This padding can contribute to the overall length of the data structure.
Packed Fields: Some languages allow you to “pack” data fields, which means they take up less space than they would otherwise. You need to factor this in when calculating the data structure’s length.
Data Type Specifics: The length of certain data types might depend on the platform. For example, a float might be 4 bytes on one platform but 8 bytes on another.
What is the date format in COBOL CopyBook?
The DATE FORMAT clause in COBOL tells the compiler that a specific data item represents a date. However, some date formats don’t explicitly state whether the day or month comes first. In these cases, the Day First setting in your Copybook connection determines the order.
But wait, there’s more! JSON, which is a popular data exchange format, uses a specific date format: YYYY-MM-DD. This means the year comes first, followed by the month, and finally the day.
Here’s a deeper dive into how COBOL handles dates:
Understanding COBOL Date Formats
COBOL offers various ways to represent dates, but you’ll encounter two main categories:
Intrinsic Date Formats: These are built-in formats recognized by the COBOL compiler. For example, “YYYYMMDD” (Year, Month, Day) and “YYMMDD” (Year, Month, Day, but with only the last two digits of the year) are intrinsic formats.
User-Defined Date Formats: These are custom formats you define using the DATE FORMAT clause. For instance, you could define a date format like “DD/MM/YYYY” or “MM-DD-YYYY”.
Day First Setting
When you use a date format that doesn’t explicitly define the order of day and month (like “MMDDYY”), the Day First setting in your Copybook connection comes into play. This setting acts like a switch.
Day First = True: This means the day comes first in the date representation (e.g., “01/01/2023” represents January 1st, 2023).
Day First = False: The month comes first (e.g., “01/01/2023” represents January 1st, 2023).
Important Note: It’s crucial to be aware of the Day First setting and the date format you use when working with COBOL dates. Misinterpretation can lead to errors in your applications.
Making It Clear:
To avoid ambiguity, it’s best practice to use intrinsic formats or explicitly define your custom formats using the DATE FORMAT clause. This ensures consistent date representation within your COBOL programs.
Let me know if you have any other questions. I’m always happy to help!
See more here: How To See Copybooks In Mainframe? | What Is A Copybook In Mainframe
What is a COBOL copybook?
Think of it this way: you’re building a program to manage customer information. You need to store things like their name, address, and phone number. A copybook would define how each of those pieces of information is structured and stored. It’s like a template, telling your program how to organize and understand the data it’s working with.
Why is this important? Well, it makes your code more organized and reusable. Instead of defining the same data structures over and over in different parts of your program, you can create a single copybook and include it wherever you need it. This helps keep your code clean, consistent, and easier to maintain.
Here’s a simple example:
“`COBOL
COPY CUSTOMER-DATA.
01 CUSTOMER-RECORD.
05 CUSTOMER-NAME PIC X(30).
05 CUSTOMER-ADDRESS PIC X(50).
05 CUSTOMER-PHONE PIC X(12).
“`
This copybook, named CUSTOMER-DATA, defines a CUSTOMER-RECORD that holds a customer’s name, address, and phone number. Each data item is given a PIC clause (picture clause) that specifies the type and size of the data. In this example, CUSTOMER-NAME is a string of up to 30 characters, CUSTOMER-ADDRESS is a string of up to 50 characters, and CUSTOMER-PHONE is a string of up to 12 characters.
Now, whenever you need to work with customer information in your COBOL program, you can simply include this copybook. It saves you from having to repeat the same data definitions over and over.
So, in a nutshell, a COBOL copybook is a powerful tool that helps you organize, reuse, and manage the data structures in your COBOL programs.
What is copybook?
A copybook is a collection of reusable code that’s stored separately from your main program. Imagine it like a set of building blocks – you can reuse these blocks in multiple programs without having to write the same code over and over again. During compilation, the mainframe compiler will “COPY” the contents of the copybook directly into your program’s source code.
So, why do we use copybooks? They bring many benefits to mainframe development:
Code Reusability:Copybooks help reduce code duplication, which means you’re writing less code overall and making your programs easier to maintain.
Modularity: By separating common code into copybooks, you can organize your codebase more effectively and make changes to common elements without touching multiple programs.
Standardization:Copybooks allow you to enforce coding standards and best practices across your projects, ensuring consistency and maintainability.
Let me give you a real-world example. Imagine you’re working on a program that needs to read and write data from a customer file. Instead of writing the code for reading and writing customer data every time you need it, you can create a copybook that contains these common functions. When you need to use them in a program, you simply use the “COPY” directive to include the copybook. This way, if you ever need to change how you interact with the customer file, you only need to modify the copybook – your program code stays the same, and you only need to recompile the program, not every single program that uses it.
Copybooks are a crucial part of the mainframe development process. They contribute to more efficient and organized code, making your work easier and more manageable.
How do I open or browse a copybook?
You can open a copybook in edit mode or read-only mode, depending on your needs.
* The Open Copy Member action lets you edit the copybook directly.
* The Browse Copy Member action lets you view it without making any changes.
* The View Copy Member action is similar to Browse Copy Member, allowing you to look at the copybook but not save any changes. However, you can always save the file under a different name if you want to make edits.
So, how do you open or browse a copybook from the COBOL Editor or System z LPEX Editor?
1. Locate the copybook: Find the copybook file you want to open.
2. Right-click on the file: This will bring up a context menu with various options.
3. Select the appropriate action: Choose Open Copy Member, Browse Copy Member, or View Copy Member depending on what you want to do.
Important Considerations:
Permissions: Make sure you have the necessary permissions to open or browse the copybook. Sometimes, you might need special access depending on the copybook’s location or security settings.
File type: Copybooks usually have a `.cpy` file extension. Make sure you are actually trying to open a copybook file.
Editor Compatibility: Ensure your editor (COBOL Editor or System z LPEX Editor) supports opening and browsing copybooks.
Let me know if you have any other questions about opening or browsing copybooks!
How many byte is a copybook?
A copybook isn’t a physical book, it’s a section of code that defines data structures in COBOL. Imagine you have a blueprint that defines the layout of a house. A copybook is like that blueprint, but for data. It tells the program how data should be organized and stored.
So, how many bytes does a copybook use? That depends on the size and complexity of the data structures it defines. A single digit in COBOL, which is stored as a packed decimal, takes up half a byte. If you have a copybook that defines a variable with 6 digits, it would use 3 bytes of storage (6 digits x 0.5 bytes/digit = 3 bytes).
But a copybook isn’t just about storing data. The real power of copybooks comes from their reusability. If you have a data structure that’s used in multiple programs, you can define it once in a copybook. Then, instead of writing the same code over and over, you can simply include the copybook into your programs using the COPY statement. This saves time and effort, and also ensures that your data structures are consistent across different parts of your application.
Think of it this way: Imagine you have a recipe for a cake. You can write that recipe down in a separate notebook (the copybook), and then you can just copy and paste that recipe into any of your cookbooks (the programs). This makes your cookbooks much easier to manage, and you always know that the cake recipe is consistent!
So, while the size of a copybook in terms of bytes can vary, the real value lies in its ability to simplify your code and make it more efficient. It’s a powerful tool for any COBOL programmer, so it’s worth learning more about how to use them effectively!
See more new information: linksofstrathaven.com
What Is A Copybook In Mainframe: A Detailed Explanation
So, you’re diving into the world of mainframes, huh? That’s cool! It’s a whole different beast compared to the regular computers we use every day. One of the things you’ll come across is something called a copybook.
Think of a copybook as a blueprint for your data. It’s a file that defines the structure of your data, kind of like a template. This means it’s a standard definition of data fields used in different programs. It lets the computer know what kind of information is stored in each field and how it’s laid out.
Why do we need copybooks?
Well, let’s say you’re working with a bunch of different programs that all need to access the same data. Without a copybook, you’d have to define the structure of your data in every single program. That’d be a huge pain, right? But with a copybook, you can define the structure once and reuse it in all your programs! It’s like having a reusable blueprint for your data.
Let’s break down the anatomy of a copybook:
– Level Number: This identifies the position of each field. The higher the level number, the more nested the field.
– Field Name: This is the name of the field that will be used to access its data.
– Data Type: This tells you what kind of data the field holds, like a number, a string of text, or a date.
– Length: This indicates the maximum size of the field.
Copybooks are a bit like building blocks
They can be combined and reused to create more complex data structures. You can create a base copybook for common data elements and then create more specific copybooks that inherit from the base. This makes it easy to manage and maintain your data definitions.
How do we use copybooks?
In a mainframe environment, copybooks are primarily used in COBOL programming. The COBOL compiler uses them to understand the structure of your data and allocate the appropriate amount of memory to hold it. It’s like giving the compiler a roadmap so it knows where everything is supposed to go.
Here’s how it works:
1. You define the copybook with all the fields and their attributes.
2. You include the copybook in your COBOL program using the COPY statement.
3. The COBOL compiler reads the copybook and uses it to define your data structures.
Now, your COBOL program can use the fields defined in the copybook to access and manipulate your data.
Let’s illustrate with a real-world example:
Imagine you’re working with a database of customer information. You could define a copybook like this:
“`
01 CUSTOMER-RECORD.
05 CUSTOMER-ID PIC 9(10).
05 CUSTOMER-NAME PIC X(30).
05 CUSTOMER-ADDRESS PIC X(50).
05 CUSTOMER-PHONE PIC X(12).
05 CUSTOMER-EMAIL PIC X(50).
“`
This copybook defines a record called CUSTOMER-RECORD. It has five fields:
– CUSTOMER-ID: A 10-digit numeric field for the customer’s ID.
– CUSTOMER-NAME: A 30-character string for the customer’s name.
– CUSTOMER-ADDRESS: A 50-character string for the customer’s address.
– CUSTOMER-PHONE: A 12-character string for the customer’s phone number.
– CUSTOMER-EMAIL: A 50-character string for the customer’s email address.
Now, any COBOL program that needs to access customer data can use this copybook. It eliminates the need to redefine the data structure in each program, making code more efficient and consistent.
Types of Copybooks:
– Standard Copybooks: These are pre-defined copybooks that come with your mainframe operating system. They provide common data structures, like date and time formats, currency conversions, and error codes.
– User-Defined Copybooks: These are custom copybooks that you create yourself to define your own data structures.
Advantages of using Copybooks:
– Reusability: You can reuse the same copybook in multiple programs, reducing redundancy and maintenance effort.
– Consistency: All programs that use the same copybook will have a consistent data structure, which helps avoid errors and makes your code easier to understand.
– Modularity: You can create separate copybooks for different parts of your data, making your code more organized and easier to manage.
– Improved Maintainability: If you need to change the data structure, you only need to update the copybook, not every program that uses it.
Copybooks are an essential part of mainframe development, providing a way to structure and manage data effectively.
In addition to COBOL, copybooks are also used in other mainframe programming languages like Assembler and PL/I.
FAQs About Copybooks
1. What are the different types of copybooks?
We already discussed the main types: Standard Copybooks and User-Defined Copybooks. Think of it as buying a pre-designed house plan (Standard Copybooks) or designing your own (User-Defined Copybooks).
2. How do I create a copybook?
You’ll need a text editor to create a copybook. It’s basically a file with special syntax that defines the data structures. You’ll find specific commands and rules in the mainframe documentation.
3. How do I use a copybook in my program?
The exact syntax for including a copybook depends on the programming language you’re using. For COBOL, you use the COPY statement.
4. Can I share copybooks across different applications?
Yes! That’s the whole point. It promotes reusability. However, ensure you have a good naming convention and version control to avoid confusion.
5. What are the best practices for using copybooks?
– Keep them organized. Don’t have a single gigantic copybook. Group related data into smaller, more manageable copybooks.
– Document them well. You (or someone else) will thank yourself later!
– Use version control. As you make changes, track versions to prevent issues.
– Use standard copybooks when possible. It’ll help with consistency and portability.
6. Are copybooks still relevant in the modern era?
Yes! Even with new technologies, copybooks continue to be used in mainframe applications. They remain a vital part of managing data effectively in this environment.
7. How do I learn more about copybooks?
You can find plenty of resources online, especially for COBOL. Look for tutorials, articles, and documentation.
Copybooks are a fundamental part of the mainframe world. They’re not as flashy as some of the newer technologies, but they’re vital for making sure data is handled correctly. So, as you dive into the world of mainframes, make sure you familiarize yourself with copybooks. They’ll be your best friend when it comes to managing data.
The COBOL copybook – IBM
A COBOL copybook is a section of code that defines the data structures of COBOL programs. Before writing your business rules, you identify the data structures on which you want to write and manage rules outside of your COBOL application. IBM
What is Copybook in COBOL? – IBM Mainframe Community
Copybooks are inserted into your program during compilation. Actually, it’s one of the compiler’s functions – insert copybooks into the source. After that, there IBM Mainframe Community
Opening, browsing, and viewing copybooks – IBM
The Open Copy Member action opens a file in edit mode. The Browse Copy Member action opens it in read-only mode. The View Copy Member action opens a copybook in edit IBM
Template and copybook concepts – IBM
A copybook is a PDS member containing either COBOL data description entries, PL/I DECLARE statements, or HLASM data description entries: COBOL copybook. Each IBM
COBOL – COPY Statement – COBOL Tutorial – IBMMainframer
COBOL – COPY Statement. The COPY statement is a library statement that places prewritten text in a COBOL compilation unit. In otherwords, COPY provides the facility to IBMMainframer Online Mainframe Tutorials
COBOL COPY Statement – www.www.mainframestechhelp.com
The COPY statement copies the copybook declarations into the module during the compilation time and logically replaces the COPY statement with its content. The Mainframestechhelp
What is copybook in detail and why we are using it
Copybook is part of code that is saved as a separate member. During compilation, this part of code is COPYed into the program’s source. For example: If IBM Mainframe Community
How To Work With Cobol Copybooks Effectively – MarketSplash
COBOL copybooks remain a crucial component for ensuring code consistency and reuse. In this article, we’ll detail their structure and usage, offering MarketSplash
What is a copybook and when should one be used? – IBM Cobol
COPYBOOKs are code that is kept in a common library (COPYlib) and is expanded in COBOL code using the COPY command. COPY is used when the same IBM Mainframe Forum
COBOL – Data Layout – Online Tutorials Library
A COBOL copybook is a selection of code that defines data structures. If a particular data structure is used in many programs, then instead of writing the same data structure TutorialsPoint
Copy Book In Cobol – Mainframe Cobol Tutorial – Part 34 #Cobol (Vol.Revised)
Copybook – Mainframe Etl Testing Tutorial – Part 8
Mainframe Cobol Copybook Mainframe New Batch Starts On Aug 31 9.30 Am |Anil Polsani |+91-9908502542
Copybook In Mainframe, Replacing Cobol, Can We Use Same Copybook Twice In A Program | Mainframe Guru
Daily Cobol #30 : Using Copybook In Cobol | Ibm Mainframe
Challenges In Copybook – Mainframe Etl Testing Tutorial – Part 10
What’S A Copybook In Mainframes?
Link to this article: what is a copybook in mainframe.
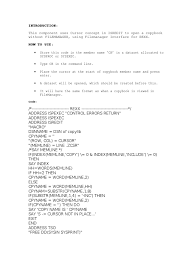
See more articles in the same category here: https://linksofstrathaven.com/how