Let’s discuss the question: java how to iterate through a treemap. We summarize all relevant answers in section Q&A of website Linksofstrathaven.com in category: Blog Finance. See more related questions in the comments below.
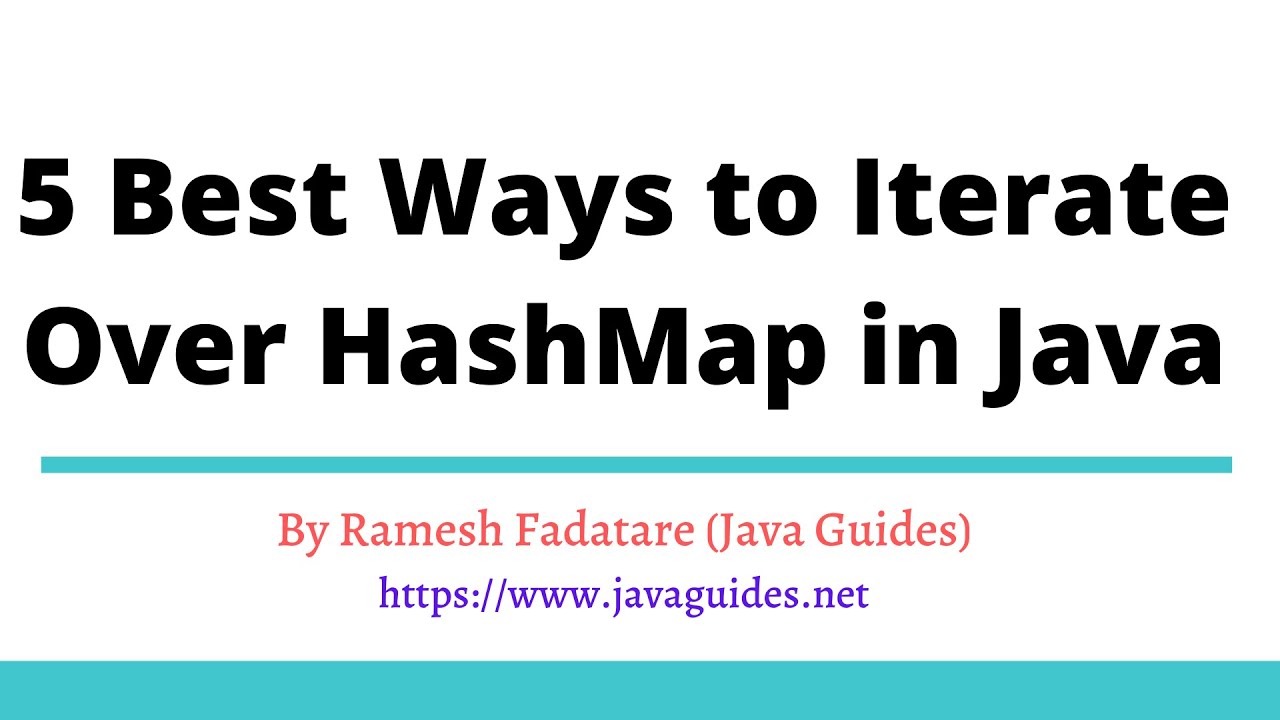
Can you iterate through a TreeMap in Java?
The TreeMap in Java is used to implement Map interface and NavigableMap along with the Abstract Class. We cannot iterate a TreeMap directly using iterators, because TreeMap is not a Collection.
How do I iterate TreeMap in order?
Using keySet(); method and for-each loop. Using keySet(); method and Iterator interface. Using entrySet(); method and for-each loop.
5 Best Ways to Iterate Over HashMap in Java
Images related to the topic5 Best Ways to Iterate Over HashMap in Java
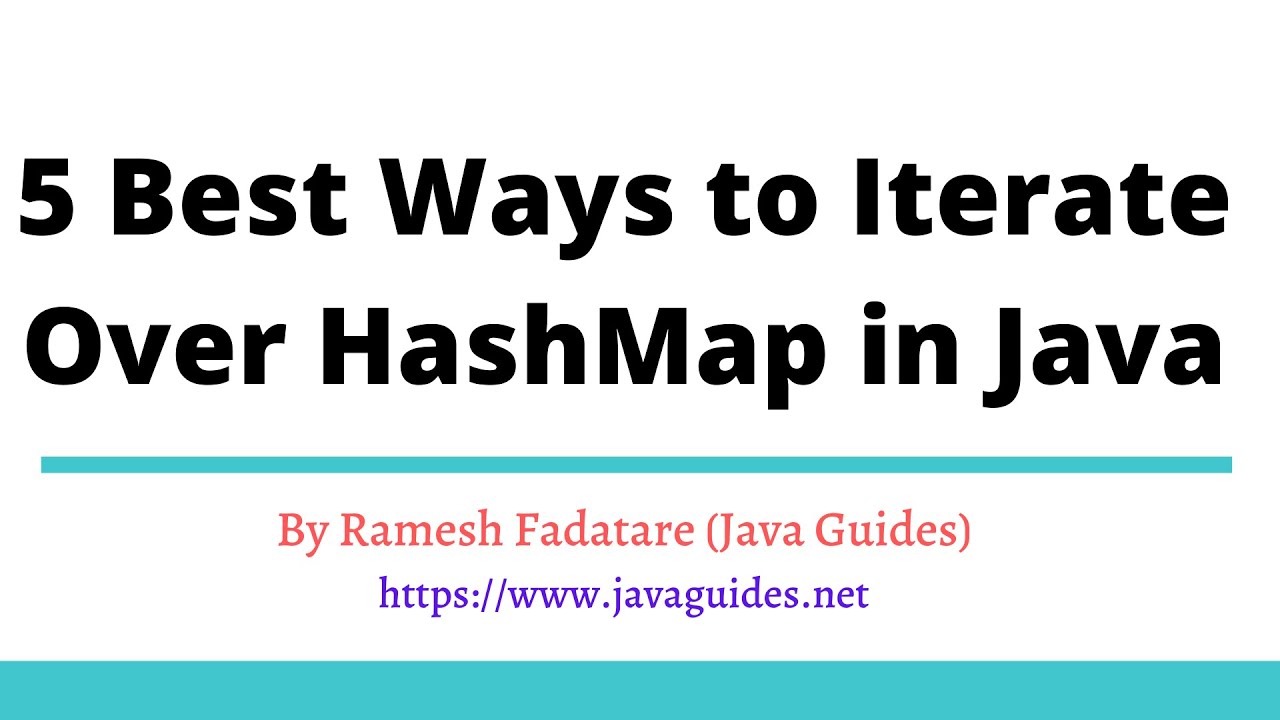
How do you iterate through a TreeSet?
- get the Iterator by calling the iterator() method.
- Use a for or while loop with hasNext()
- Call the next() method.
How do I iterate TreeMap in reverse order?
- Using the reverseOrder() method.
- Using the descendingKeySet() method.
- Using the descendingMap() method.
Is TreeMap sorted?
TreeMap is a map implementation that keeps its entries sorted according to the natural ordering of its keys or better still using a comparator if provided by the user at construction time.
Can we iterate HashMap?
There is a numerous number of ways to iterate over HashMap of which 5 are listed as below: Iterate through a HashMap EntrySet using Iterators. Iterate through HashMap KeySet using Iterator. Iterate HashMap using for-each loop.
What is iterator in Java?
An Iterator is an object that can be used to loop through collections, like ArrayList and HashSet. It is called an “iterator” because “iterating” is the technical term for looping. To use an Iterator, you must import it from the java. util package.
Is TreeMap entrySet sorted?
As you can see, TreeMap defines an inner class called TreeMap. EntrySet which just extends AbstractSet. And no, it does not implement SortedSet (which would otherwise probably be specified by the SortedMap.
What does entrySet do in Java?
entrySet() method in Java is used to create a set out of the same elements contained in the hash map. It basically returns a set view of the hash map or we can create a new set and store the map elements into them.
How do I add a comparator to TreeSet in Java?
- import java.util.Comparator;
- import java.util.TreeSet;
- public class JavaTreeSetComparatorExample2 {
- public static void main(String a[]){
- TreeSet <String> obj = new TreeSet<String>();
- obj.add(“B”);
- obj.add(“b”);
- obj.add(“A”);
What is SortedSet in Java?
SortedSet , is a subtype of the java. util. Set interface. The Java SortedSet interface behaves like a normal Set with the exception that the elements it contains are sorted internally. This means that when you iterate the elements of a SortedSet the elements are iterated in the sorted order.
What is TreeSet and HashSet in Java?
A TreeSet is a set where the elements are sorted. A HashSet is a set where the elements are not sorted or ordered. It is faster than a TreeSet. The HashSet is an implementation of a Set.
Java Tutorial – Using TreeMap
Images related to the topicJava Tutorial – Using TreeMap

How do I print TreeMap?
- for (Map. Entry<K, V> entry : myMap. entrySet()) {
- System. out. println(“Key: ” + entry. getKey() + “. Value: ” + entry. getValue());
- }
How do I convert a map to TreeMap?
…
Algorithm:
- Get the HashMap to be converted.
- Create a new TreeMap using Maps. …
- Pass the hashMap to putAll() method of treeMap.
- Return the formed TreeMap.
What is difference between HashMap and TreeMap?
HashMap allows a single null key and multiple null values. TreeMap does not allow null keys but can have multiple null values. HashMap allows heterogeneous elements because it does not perform sorting on keys. TreeMap allows homogeneous values as a key because of sorting.
Does TreeMap sort by keys?
A TreeMap is always sorted based on keys. The sorting order follows the natural ordering of keys. You may also provide a custom Comparator to the TreeMap at the time of creation to let it sort the keys using the supplied Comparator.
How do I sort objects in TreeMap?
To sort keys in TreeMap by using a comparator with user-defined objects in Java we have to create a class that implements the Comparator interface to override the compare method. In the below code, we are passing a custom object as a key in TreeMap i.e Student user-defined class.
Is TreeMap an interface?
The TreeMap in Java is used to implement Map interface and NavigableMap along with the AbstractMap Class. The map is sorted according to the natural ordering of its keys, or by a Comparator provided at map creation time, depending on which constructor is used.
How many ways we can iterate Map in Java?
There are generally five ways of iterating over a Map in Java.
What is the best way to iterate HashMap in Java?
- Using enrtySet() in for each loop. for (Map.Entry<String,Integer> entry : testMap.entrySet()) { …
- Using keySet() in for each loop. for (String key : testMap.keySet()) { …
- Using enrtySet() and iterator. …
- Using keySet() and iterator.
Can you iterate over elements stored in Java HashMap?
HashMap iteration with forEach()
In the first example, we use Java 8 forEach method to iterate over the key-value pairs of the HashMap . The forEach method performs the given action for each element of the map until all elements have been processed or the action throws an exception.
How do you iterate in Java?
- Obtain an iterator to the start of the collection by calling the collection’s iterator() method.
- Set up a loop that makes a call to hasNext(). Have the loop iterate as long as hasNext() returns true.
- Within the loop, obtain each element by calling next().
Different Ways to Iterate Through a Map in Java
Images related to the topicDifferent Ways to Iterate Through a Map in Java

How do I use an iterator in Java?
- Obtain an iterator to the start of the collection by calling the collection’s iterator( ) method.
- Set up a loop that makes a call to hasNext( ). Have the loop iterate as long as hasNext( ) returns true.
- Within the loop, obtain each element by calling next( ).
How Java iterator works internally?
The iterator for most simple java collections just keeps a pointer of where in the collection the iterator is currently at. Calling . next() will advance the iterator. It doesn’t copy the elements, and just returns the next element from the collection.
Related searches
- java treemap
- how to iterate through a tree
- how to iterate through a treeset in java
- how does a treemap work in java
- best way to iterate over map in java
- Iterator TreeMap java
- how to loop through a treemap in java
- for map java
- treemap foreach java 8
- get key in treemap java
- iterator treemap java
- Treemap put
- For (Map Java)
- java stream list to treemap
- best way to iterate hashmap
- reverse treemap java
- treemap put
- how to use iterator for treemap in java
Information related to the topic java how to iterate through a treemap
Here are the search results of the thread java how to iterate through a treemap from Bing. You can read more if you want.
You have just come across an article on the topic java how to iterate through a treemap. If you found this article useful, please share it. Thank you very much.