What does Cannot be dereferenced mean in Java?
When you get the error “X cannot be dereferenced”, it means you’re trying to use a reference to access an object, but the reference doesn’t point to an object. The error message tells you that you’re trying to unlock a door, but you don’t have a key.
Primitive data types in Java are not objects. They’re simply values that are stored directly in memory. Think of them like a single piece of furniture, not an entire room. You can’t use a reference to access a primitive data type because there’s no object to access.
Here’s an analogy: Imagine you have a box with a label saying “Apple”. The box itself is the reference, and the “Apple” inside is the object. If you try to open the box and see what’s inside, you’re dereferencing the reference.
Now imagine you have a box labeled “Red”. The color “Red” is a primitive type. You can’t open the box and find something called “Red” inside. It’s just a color, not an object.
So, the error “X cannot be dereferenced” tells you that you’re trying to open a box that doesn’t have an object inside. It’s like trying to open a box labeled “Red” and expecting to find something called “Red” inside—you’re not going to find anything because it’s not an object.
Let’s look at some Java code examples to make this clearer.
“`java
int num = 5; // num is a primitive type, not an object
num.toString(); // This will throw a “Cannot be dereferenced” error
“`
In this example, we are trying to call the `toString()` method on the `num` variable, which is an `int` (an integer). Since `int` is a primitive type, it doesn’t have any methods or properties associated with it, so we cannot dereference it.
However, if we use a wrapper class like `Integer`, it becomes an object.
“`java
Integer num = 5; // num is now an object
num.toString(); // This will work fine, as num is now an object
“`
This example shows that the `Integer` class is a wrapper class for the primitive type `int`. It allows us to treat primitive types as objects. We can now call methods like `toString()` on `num` because it is now an object.
In summary, the error “X cannot be dereferenced” means that you are trying to access an object through a reference that does not point to an object. This error usually occurs when you are trying to use a method or property on a primitive data type. To fix this error, you need to ensure that you are using a reference that points to an actual object.
What is meant by dereference in Java?
Imagine you have a variable that holds the address of a location in memory. Think of this variable as a pointer, pointing to that specific location. To access the actual data stored at that address, you need to dereference the pointer. In Java, you do this using the asterisk symbol (*).
Let’s look at an example:
“`java
int *ptr = # // Declare a pointer and assign the address of the variable ‘num’ to it.
int value = *ptr; // Dereference the pointer to get the value stored at the memory address it points to.
“`
In this example, `ptr` acts as a pointer, storing the memory address of the variable `num`. By using `*ptr`, we dereference the pointer and retrieve the value stored at the address it points to. We can then store this value in another variable, `value`.
Dereferencing allows you to directly manipulate the data stored in memory. This is a core concept in programming, enabling you to work with dynamically allocated memory, create linked lists, and perform various other operations. Understanding dereferencing is essential for mastering concepts like pointers and dynamic memory allocation in Java, which can significantly enhance your programming abilities.
How to avoid null dereference in Java?
While Java doesn’t *prevent* null pointers, we have some awesome tools to help us catch those sneaky nulls before they cause problems. The first rule of thumb: always check for null before you use an object. It’s like double-checking your keys before you leave the house—you want to be sure you’re working with something real.
Here’s a simple example:
“`java
String name = null;
if (name != null) {
System.out.println(“My name is: ” + name);
} else {
System.out.println(“I don’t have a name yet.”);
}
“`
In this code, we check if the variable `name` is null before we try to print it. This way, we avoid that nasty null pointer exception and instead print a friendly message.
But checking for null every time can get tedious, right? Thankfully, Java offers some neat features to help us out:
Optional: This handy class from Java 8 is like a wrapper for your potentially null values. It’s a great way to handle nulls gracefully and avoid cluttering your code with tons of `if` statements.
Null-safe operators: Java 11 introduced these cool operators (`?.` and `?:`) to make null checks even easier. The `?.` operator lets you access a field or method only if the object is not null. The `?:` operator lets you provide a default value if the object is null.
Let’s see an example with the Optional class:
“`java
Optional
if (name.isPresent()) {
System.out.println(“My name is: ” + name.get());
} else {
System.out.println(“I don’t have a name yet.”);
}
“`
In this example, we use the `Optional.ofNullable()` method to create an Optional object. Then, we can use the `isPresent()` method to check if the Optional object contains a value. If it does, we can use the `get()` method to retrieve the value.
So, keep these techniques in mind and say goodbye to those pesky null pointer exceptions!
How to see if two chars are equal in Java?
Let’s break down how this works. In Java, Character is a wrapper class that allows you to treat a single character as an object. This lets you use methods like equals() to compare characters, which you can’t directly do with primitive `char` data types.
So, when you use the equals() method, you’re basically checking if the underlying `char` values of the two Character objects are the same. The equals() method, in its core, does a simple comparison of the `char` values.
Here’s a simple example:
“`java
Character char1 = ‘A’;
Character char2 = ‘A’;
boolean areEqual = char1.equals(char2); // This will be true
Character char3 = ‘B’;
boolean areNotEqual = char1.equals(char3); // This will be false
“`
In the example above, we create two Character objects, char1 and char2, both holding the character ‘A’. We then compare them using equals() and store the result in areEqual. Since both objects hold the same character, areEqual will be true.
We then create a third Character object, char3, holding the character ‘B’. When we compare char1 and char3, the result is stored in areNotEqual. As the characters are different, areNotEqual becomes false.
This simple comparison is the core of what the equals() method does. It’s crucial for ensuring accurate comparisons between characters when working with the Character class in Java.
What is a null dereference in Java?
Imagine you have a variable called “myCar.” This variable is supposed to hold information about your car, like its color or model. But what if “myCar” doesn’t actually have any car information assigned to it? In this case, “myCar” is null.
Now, if you try to access information from “myCar,” like trying to get the car’s color, you’re attempting a null dereference. This is like trying to open a door that isn’t there—your program will crash, and you’ll get an error.
Here’s an example:
“`java
String carColor = myCar.getColor(); // myCar is null, so this will cause a null dereference
“`
Why is this a problem? Well, null dereference is one of the main culprits behind program crashes. It’s often tricky to detect because you might not realize that a variable is null until you try to use it.
Fortunately, Java has tools to help you avoid this problem. Null checks are a simple way to ensure that a variable is not null before you use it. This way, you can gracefully handle the situation instead of crashing your program.
So, remember, null dereference happens when you try to use a variable that’s null (doesn’t point to anything). Always be careful about your variables and check for null values before using them!
Why is it called dereference?
Think of it this way: Imagine you have a map that shows you where a treasure is buried. The map is like a pointer, and the treasure itself is the object. You can’t get the treasure just by holding the map; you need to use the map to dereference it and find the treasure.
The “de-” prefix in dereference likely comes from the Latin preposition “de,” which means “from.” So, dereferencing a pointer can be seen as “obtaining the object from the reference,” just like using a map to find a treasure.
Let’s look at a simple example: Imagine a variable named “my_number” that stores the value 5. Now, let’s create a pointer named “pointer_to_my_number” and point it to “my_number.” When we dereference “pointer_to_my_number,” we’re essentially saying, “Go to the memory location pointed to by this pointer and get the value stored there.” This would give us the value 5, which is stored in the variable “my_number.”
In programming languages like C and C++, the dereference operation is usually represented by an asterisk (*). So, to dereference “pointer_to_my_number,” we would write “*pointer_to_my_number.” This would then give us the value 5.
Understanding dereferencing is crucial for working with pointers, which are fundamental tools for managing memory in many programming languages. It allows you to access and manipulate the data stored at a specific memory location, providing a powerful way to work with your program’s data.
See more here: What Is Meant By Dereference In Java? | Double Cannot Be Dereferenced Equals
Can a double error be dereferenced?
Let’s dive into why “Double cannot be dereferenced” occurs and why it’s crucial to understand the difference between primitive types and reference types in Java.
In Java, primitive types like double store actual values directly. Think of them as simple containers holding a number. On the other hand, reference types like String or objects hold references to where the actual data is stored in memory.
Dereferencing means accessing the data pointed to by a reference. When you dereference a reference, you’re essentially saying, “Go to this memory location and get me the value stored there.”
Doubles, however, are not references. They are primitive types, meaning they hold the value directly. So, trying to dereference a double is like trying to open a door that doesn’t exist – it simply doesn’t make sense!
Java’s compiler is smart enough to catch this error and throws the “Double cannot be dereferenced” exception. This is a safety mechanism to prevent potential bugs and crashes.
To avoid this error, you need to ensure you’re dealing with reference types if you intend to dereference them. For double values, you can perform arithmetic operations directly on the variable itself.
Remember, understanding the distinction between primitive types and reference types is fundamental to writing correct and efficient Java code.
Can double be dereferenced?
Let’s delve deeper into why double can’t be dereferenced:
In Java, dereferencing means accessing the value stored in a reference variable. A reference variable holds the memory address of an object. Primitive data types, like double, do not have memory addresses, but rather hold their actual value directly. Therefore, you can’t dereference a double because it doesn’t represent an object’s location in memory.
Let’s consider an analogy to illustrate this. Imagine you have a box (the reference variable) that contains a key (the memory address). The key unlocks a room (the object) where a treasure (the value) is stored. You can use the key to access the treasure, which is analogous to dereferencing a reference.
However, if you have a double, it’s like having a treasure chest (the double variable) containing the treasure (the value) directly, without any key or room involved. You can directly access the treasure inside the chest without a key, as there’s no room to unlock.
In essence, double is a fundamental data type that stores its value directly, eliminating the need for dereferencing. If you’re encountering issues related to double, it’s important to carefully analyze the context and ensure you’re using the correct operations and understanding its inherent characteristics.
Can a naming convention double be dereferenced?
Java compilers give this error when you try to call a method on a primitive double type. double is a primitive data type, meaning it represents a fundamental value, like a number. In contrast, objects have methods and properties. Primitives don’t have methods in Java.
Let’s break it down:
Primitives are basic data types, like int, double, char, and boolean. They directly hold values.
Objects are instances of classes, which contain methods and properties.
Since double is a primitive, you cannot directly call a method on it. Java’s error message is trying to explain that you’re attempting to treat a primitive like an object.
Here’s a simple example:
“`java
double myDouble = 3.14;
myDouble.toString(); // This will cause the error: “double cannot be dereferenced”
“`
The toString() method is a method of the Double class, which is the object wrapper class for the primitive double. To use toString() on a double, you need to wrap it in the Double class:
“`java
double myDouble = 3.14;
Double doubleObject = new Double(myDouble); // Wrap the double as a Double object
String myString = doubleObject.toString(); // Now you can use the toString() method
“`
Remember, double is a primitive, so treat it as such. Use the Double class when you need to access methods and properties associated with double values.
Why am I getting a ‘double’ error?
You’re seeing this error because double is a primitive data type in Java, not an object. This means it doesn’t have any methods associated with it, like toString().
To fix this, you need to use the Double wrapper class. Instead of `price.toString()`, use `Double.toString(price)`. This will convert the double value to a String.
Understanding the Difference Between Primitive Types and Wrapper Classes
In Java, primitive types like double, int, float, char, and boolean represent basic data values. They are directly stored in memory and don’t have any associated methods. On the other hand, wrapper classes like Double, Integer, Float, Character, and Boolean are objects. They provide methods that allow you to manipulate and work with primitive data types in a more object-oriented way.
For example, if you want to convert a double value to a string, you need to use the Double wrapper class. You can’t call the toString() method directly on a double value, as it doesn’t have this method. Instead, you use the Double.toString() method, which takes a double value as input and returns its string representation.
Here’s a simple example to illustrate this:
“`java
double price = 10.99;
// Using the Double wrapper class to convert the double value to a string
String priceString = Double.toString(price);
// Output: 10.99
System.out.println(priceString);
“`
Remember, using the wrapper classes gives you access to a wider range of methods for manipulating primitive data types. So, whenever you need to perform operations on primitive types, make sure to use the corresponding wrapper class to leverage these functionalities.
See more new information: linksofstrathaven.com
Double Cannot Be Dereferenced Equals: Understanding The Error
Imagine you have a box, and you want to put something inside. You can’t just put the box itself inside another box, right? You need to open the box, get the contents, and then put those contents into the other box.
That’s kind of how it works with variables in programming. Some variables are like containers, holding values you can access directly. Others are like boxes, holding references to other things – references that can be used to access the actual values they point to.
In Java, `double` is a primitive data type, meaning it directly holds a value. This value could be a number like 3.14159 or -1.25. It’s not a box with a reference.
The error “double cannot be dereferenced” happens when you try to treat a `double` variable as a box that holds a reference. You’re trying to get the contents of something that’s not a reference.
Let’s use a simple example:
“`java
double myNumber = 5.0;
myNumber.equals(3.0); // This will cause the error!
“`
Here, `myNumber` is a `double` variable. It holds the value 5.0 directly. We’re trying to use the `equals` method, which is intended for comparing objects. But a `double` variable is not an object! It’s just a plain value.
How to Solve this Error
You can solve this error by understanding that you can’t dereference a `double` because it’s not an object. The most common way to compare `double` values is to use the `==` operator:
“`java
double myNumber = 5.0;
if (myNumber == 3.0) {
// Code to execute if myNumber equals 3.0
}
“`
Remember:
* `double` is a primitive data type, it doesn’t have methods.
* `==` operator compares the actual values of two `double` variables.
* Always use the `==` operator to compare `double` values for equality.
If you’re getting this error and you’re sure you’re dealing with a `double` variable, check your code carefully. Make sure you’re not trying to use methods or perform actions meant for objects on a `double` variable. And if you’re confused, feel free to ask me, I’m here to help.
Common Situations and Solutions
Let’s look at some scenarios where this error might pop up and how you can fix them:
1. Trying to Access a Method on a `double` Variable:
“`java
double myNumber = 5.0;
myNumber.toString(); // This will cause the error!
“`
Solution: `double` values are primitive data types and don’t have methods like `toString()`. Use `Double.toString(myNumber)` to convert the `double` to a `String`.
2. Comparing `double` Values for Equality:
“`java
double myNumber1 = 3.14;
double myNumber2 = 3.14;
if (myNumber1.equals(myNumber2)) { // This will cause the error!
// Code to execute if myNumber1 equals myNumber2
}
“`
Solution: `double` values don’t have the `equals` method. Use the `==` operator to check if two `double` values are equal.
3. Using a `double` as a Key in a Map:
“`java
Map
myMap.put(3.14, “Pi”); // This will cause an error!
“`
Solution: `double` values cannot be used directly as keys in maps. You need to use a wrapper class like `Double`. Instead of `myMap.put(3.14, “Pi”)`, use `myMap.put(new Double(3.14), “Pi”)`.
FAQs:
Q: What is the difference between `double` and `Double`?
A: `double` is a primitive data type that directly holds a numerical value. `Double` is a wrapper class that represents a `double` value as an object. You can use the `Double` class to provide methods and functionalities that are not directly available for `double` primitives.
Q: When should I use `double` versus `Double`?
A: Use `double` when you need a simple, efficient way to store and manipulate numerical values. Use `Double` when you need to treat a number as an object, to use object-oriented features like methods, to store it in collections like lists and maps, or when you need to handle null values.
Q: Can I compare `double` values using `equals()` directly?
A: No, you can’t directly use `equals()` to compare `double` values. It’s designed for objects, not primitive data types. Use the `==` operator for `double` value comparisons.
Q: Is `==` always reliable for comparing `double` values?
A: Be careful! While `==` is the correct way to compare `double` values for equality, remember that `double` values can sometimes have rounding errors due to the way computers represent floating-point numbers. In cases where extreme precision is needed, consider using a library that provides more robust comparison methods for floating-point values.
Remember, the world of programming is full of complexities. Understanding the nuances of data types and their differences can be crucial to writing efficient and error-free code. If you’re ever unsure, don’t hesitate to ask for help. Happy coding!
How to fix “double cannot be dereferenced” in Java
Im trying to take doubles and double arrays as parameters for my methods, but when Im calling the methods I come up with the error, “double cannot be dereferenced”. I’ve tried different syntaxes, such as the var.method (array []); , Stack Overflow
java – Double cannot be dereferenced? – Stack Overflow
ORIGINAL ANSWER (addressed a different problem in your code): In double hours = Mins / 60; you are dividing two int s. You will get the int value of that Stack Overflow
java – Double cannot be dereferenced…? – Stack Overflow
Consider using the Double wrapper class like this. new Double(rec1.getWidth()).compareTo(rec2.getWidth()); Only the first value need to be Stack Overflow
Double cannot be dereferenced: causes and solutions
Double cannot be dereferenced is a compiler error that occurs when you try to access the value of a double variable using the * operator. This error occurs hatchjs.com
Java “int/char Cannot Be Dereferenced” Error | Baeldung
Take a closer look at the Java error “int cannot be dereferenced”, learn the leading cause of the exception, and see how to fix it. Baeldung
Double cannot be dereferenced
The compiler message (“double cannot be dereferenced”) is telling you that you can’t do this because doubles don’t have any methods. Why did you abandon the javaprogrammingforums.com
[Java] I keep getting the “double cannot be dereferenced … – Reddit
I keep receiving the “double cannot be dereferenced” error when I tried to compile my program. It occured at the ‘else if (!doubleAmount.hasNextDouble ())’. I don’t really know Reddit
double cannot be dereferenced (Beginning Java forum at
I think you’re getting the error because the primitive types – including ‘double’ – are not objects; they cannot have members, and in particular, don’t have a toString() member Coderanch
How to Fix int cannot be dereferenced Error in Java?
Here, we will follow the below-mentioned points to understand and eradicate the error alongside checking the outputs with minor tweaks in our sample code. GeeksForGeeks
Double cannot be dereferenced – Coderanch
If I have a Date object, pointed to by variable d, I can call d.setTime (). String does not have such a method, so if I have String variable s, I cannot call s.setTime (). So, if your tic () Coderanch
Error: Cannot Be Dereferenced (Java Tutorial)
Java 34 . Hiểu Rõ Phương Thức So Sánh Equals Và Hascode | Phần 2 – Lập Trình Hướng Đối Tượng
Dereferencing In C
New \U0026 Delete Operators For Dynamic Memory Allocation | C++ Tutorial
Error: Incompatible Types Int Cannot Be Converted To Boolean
How To Fix The Error \”Cannot Invoke The Compareto() On Primitive Type Int\” With Integer.Compare(X,Y)
C Programming: What Does Dereferencing A Pointer Mean?
Link to this article: double cannot be dereferenced equals.
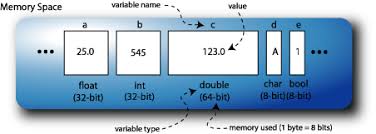
See more articles in the same category here: https://linksofstrathaven.com/how