How do you catch an index out of bounds in Java?
Inside the catch block, you can do a few things. You might want to print a message to the console to let the user know something went wrong. Or, you could log the error to a file for later analysis. You can even choose to implement a recovery strategy, like prompting the user to enter valid input. This all depends on the specific requirements of your application.
Let’s take a closer look at the try-catch structure. Here’s a basic example:
“`java
try {
// Code that might throw ArrayIndexOutOfBoundsException
int[] myArray = {1, 2, 3};
System.out.println(myArray[4]); // This will cause the exception
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Error: Array index out of bounds.”);
}
“`
In this example, the code tries to access an element at index 4 in the `myArray` which only has three elements. This throws the `ArrayIndexOutOfBoundsException`. The catch block catches the exception and prints a helpful error message to the console.
Remember, try-catch blocks are not just for catching exceptions. They also help improve the robustness of your code. By catching exceptions and handling them gracefully, you make your program more reliable and less prone to crashing.
How to avoid index out of bounds Java?
To keep your code smooth and error-free, the key is proper validation. This means checking the index before you use it to access an element in your array or list.
Think of it like this: Imagine you have a bookshelf with 5 shelves, numbered 1 through 5. If you try to grab a book from shelf number 6, you’ll end up empty-handed! You need to make sure the shelf number is within the valid range (1 to 5).
Let’s look at some code examples:
“`java
// Example 1: Validating before accessing
int[] myArray = {1, 2, 3, 4, 5};
int index = 3;
if (index >= 0 && index < myArray.length) {
// Access the element at the given index
int value = myArray[index];
System.out.println("Element at index " + index + ": " + value);
} else {
// Handle the invalid index
System.out.println("Invalid index! Make sure the index is within the array bounds.");
}
// Example 2: Using enhanced for loops
for (int element : myArray) {
System.out.println(element);
}
```
In the first example, we use an `if` statement to check if the `index` is valid before accessing the element. The second example uses an enhanced for loop to iterate through the array without worrying about index management.
Enhanced for loops are your best friend when dealing with arrays or collections because they automatically handle the iteration without you needing to manage the index manually, reducing the risk of errors. It's like having an assistant who takes care of the book-grabbing process for you, ensuring you only get books from existing shelves.
Remember, preventing index out of bounds errors is essential for writing robust and reliable Java code. By always validating your indexes and leveraging enhanced for loops when appropriate, you can ensure your code runs smoothly and avoid these common pitfalls.
How to handle ArrayIndexOutOfBoundsException in Java using try catch?
You can use a `try-catch` block to prevent your program from crashing when an `ArrayIndexOutOfBoundsException` occurs. This is a common exception that happens when you try to access an array element that doesn’t exist. The idea is to “catch” the exception and handle it gracefully.
Imagine you have a basket of apples and want to take out the 10th apple, but there are only 5 apples. This is like trying to access an element beyond the bounds of your array! The `try-catch` block allows you to anticipate and handle this situation.
Here’s a breakdown:
`try`: This block contains the code that might potentially throw an `ArrayIndexOutOfBoundsException`.
`catch`: This block catches the exception if it occurs. You can then provide a custom response, such as printing a message to the user, handling the situation differently, or logging the error for later analysis.
Let’s see it in action:
“`java
int[] numbers = {1, 2, 3, 4, 5};
try {
System.out.println(numbers[5]); // Trying to access an element beyond the array bounds!
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Oops! You tried to access an element that doesn’t exist. Please be careful.”);
// Here, you can add more specific handling, like suggesting alternative actions or logging the error
}
“`
In this example, the `try` block attempts to print the 6th element (`numbers[5]`) in the `numbers` array. Since the array only has 5 elements, an `ArrayIndexOutOfBoundsException` is thrown. The `catch` block catches the exception and prints a friendly message to the user, informing them about the issue.
You’ve now learned how to handle the exception! You can customize your `catch` block to suit the specific needs of your program. Remember, `try-catch` allows you to anticipate potential issues, gracefully handle them, and keep your code from crashing!
What do you mean by array index out of bounds exception and null pointer exception?
Null pointer exception occurs when you try to access a variable that hasn’t been assigned a value, or in other words, it’s pointing to “nothing.” Think of it like trying to use a remote control with no batteries – it’s not going to do anything. Similarly, a null pointer is like a broken remote – it doesn’t point to anything useful, and any attempt to use it will cause an error.
Array index out of bounds exception happens when you try to access an element in an array using an index that’s beyond the array’s valid range. Imagine you have a shelf with 10 books, numbered 1 through 10. If you try to grab the 12th book, you’ll find there’s nothing there! The same applies to arrays – they have a defined size, and trying to access a nonexistent element throws an index out of bounds exception.
Here’s a real-life scenario to illustrate: imagine you’re creating a shopping list for your grocery trip. Each item on your list corresponds to an element in an array. If you accidentally write down the number “12” on your list, but you only have 10 items, you’ll get an index out of bounds exception when you try to access that non-existent item.
To avoid these exceptions, we need to be careful about how we use variables and arrays. Always initialize your variables before using them, and double-check the size of your arrays before trying to access elements beyond their boundaries. Remember, it’s always a good idea to be cautious with your code and avoid these errors!
How to fix list index out of range?
The key is to make sure you’re working within the bounds of your list. Think of it like a row of seats in a theater – if you try to sit in a seat that’s not there, you’ll get an error. To avoid this, you can use the `len()` function to figure out the total number of seats (elements) in your list. Then, use the `range()` function to create a safe zone for accessing your list elements.
For example, let’s say you have a list called `fruits` with these elements: “apple”, “banana”, “cherry”. This list has three elements, so its `len()` is 3. You can use `range(3)` to safely access these elements. Here’s how you can access these elements with their indices:
“`python
fruits = [“apple”, “banana”, “cherry”]
for i in range(len(fruits)):
print(fruits[i])
“`
This code snippet iterates through each index from 0 to 2 (the length of the list minus 1) and prints out the corresponding element. This ensures you are always within the bounds of your list, preventing the dreaded “IndexError”.
Now, let’s dive a bit deeper into why this happens and how to debug it.
The “IndexError: list index out of range” occurs when your code tries to access an element using an index that’s outside the valid range of your list. Lists in Python are zero-indexed, meaning the first element has an index of 0, the second element has an index of 1, and so on. If you try to access an element with an index equal to or greater than the length of your list, you’ll encounter this error.
Let’s say you have a list with four elements. The valid indices for this list are 0, 1, 2, and 3. If your code attempts to access an element at index 4 (which doesn’t exist), you’ll get the “IndexError”.
Here are some scenarios where you might encounter the “IndexError”:
Trying to access an element beyond the last index: If your list has five elements, the valid indices are 0, 1, 2, 3, and 4. Trying to access the element at index 5 will cause the error.
Using a negative index without checking the list length: Negative indices are used to access elements from the end of the list. For example, `fruits[-1]` accesses the last element. However, if you use a negative index that’s greater than the length of the list, you’ll also encounter the “IndexError”.
Iterating through a list without considering the size: If you’re using a loop to access elements in a list, make sure your loop doesn’t exceed the length of the list.
To prevent this error, always be mindful of the size of your list and use the `len()` function to determine the valid range of indices.
How to solve string index out of bounds exception in Java?
Now, let’s dive a bit deeper. Think of a String like a row of houses with numbered addresses. Each character in your String has its own address, starting from zero. When you use charAt(index), you’re requesting the character at a specific address. But, what happens if you ask for a character that’s beyond the last house’s address? That’s when the StringIndexOutOfBoundsException pops up!
For instance, let’s say you have a String named “Hello”. The addresses would look like this:
H – address 0
e – address 1
l – address 2
l – address 3
o – address 4
If you use charAt(5), you’re essentially asking for the character at address 5. But, there’s no house at that address! This is why you’ll get the StringIndexOutOfBoundsException.
To prevent this, you can do a few things:
1. Double-check your indexes: Ensure your code uses valid indexes, staying within the bounds of your String’s length. Use the length() method to figure out the highest valid address.
2. Use the try-catch block: Wrap your code that might potentially throw the exception inside a try-catch block. The catch block will handle the exception gracefully, preventing your program from crashing.
3. Provide clear error messages: In the catch block, give users a helpful message that explains why the exception occurred. For example, you could say “Invalid input: Index out of bounds. Please check your input.”
Remember, understanding the concept of string indexes and using proper error handling practices will make your Java code more robust and user-friendly!
See more here: How To Avoid Index Out Of Bounds Java? | Java Try Catch Index Out Of Bounds
What is an array index out of bounds exception?
Think of it this way: each element in an array has a unique address, like a house number. When you try to access an element at a non-existent address, your code goes looking for a house that simply isn’t there. The compiler, acting like the friendly neighborhood mail carrier, can’t find the address and throws an exception to let you know there’s a problem.
This exception is common, especially when you’re working with loops and iterating through arrays. To prevent this error, you need to make sure that the index you’re using to access an element is within the array’s bounds. You can do this by carefully checking the loop conditions and making sure they don’t go beyond the limits of your array.
Here are a couple of examples of how an Array Index Out of Bounds Exception could occur:
Accessing an element at a negative index: For example, if you have an array called `numbers` with the values `[1, 2, 3]`, and you try to access `numbers[-1]`, you’ll get an Array Index Out of Bounds Exception. There’s no element at index -1.
Accessing an element at an index greater than or equal to the length of the array: In the same `numbers` array, trying to access `numbers[3]` (which is equal to the length of the array) will also cause an exception. There is no fourth element in this array.
These are just two examples, but the principle remains the same. If you find yourself facing an Array Index Out of Bounds Exception, look at the code that’s accessing the array and make sure the indexes are within the array’s bounds. It’s like making sure you’re visiting the right house!
What is indexoutofboundsexception in Java?
The IndexOutOfBoundsException is part of the `java.lang` package, which means it’s a fundamental part of the Java language. It’s a runtime exception, meaning it won’t be caught by the compiler and will only show up when your program actually runs.
Imagine an array like a row of seats in a theater. Each seat has a number, starting from 0. If the theater has 10 seats, the valid seat numbers are 0 to 9. If you try to access a seat with number 10 or higher, you’ll get an IndexOutOfBoundsException!
Here’s a simple example to illustrate:
“`java
int[] numbers = {1, 2, 3, 4, 5};
// This will cause an IndexOutOfBoundsException because
// the valid indices are 0 to 4.
int number = numbers[5];
“`
Let’s break it down:
* `numbers` is an array that holds the values 1, 2, 3, 4, and 5.
* The valid indices for accessing these numbers are 0, 1, 2, 3, and 4.
* When we try to access `numbers[5]`, we’re asking for the element at index 5, which is beyond the array’s bounds. This throws the IndexOutOfBoundsException.
Remember, in Java, arrays are zero-indexed, meaning the first element is at index 0.
Understanding IndexOutOfBoundsException is important for writing robust and error-free Java code.
How to avoid arrayindexoutofboundsexception in Java?
Let’s break this down with a simple example. Imagine you have an array named `myNumbers` that holds five integers.
“`java
int[] myNumbers = { 10, 20, 30, 40, 50 };
“`
In this case, `myNumbers.length` would be 5, but the valid indices to access elements in this array are 0, 1, 2, 3, and 4. If you try to access an element at index 5 or beyond, you’ll get that dreaded `ArrayIndexOutOfBoundsException` – Java’s way of saying “Hey, you’re trying to access something that doesn’t exist!”
Always remember to check the array’s length before accessing any element. Use `arrayName.length` to get the length of the array. This will help prevent the dreaded ArrayIndexOutOfBoundsException.
Remember:
Index 0: The first element of your array is always found at index 0.
Index (length – 1): The last element is at the index one less than the length of the array.
By understanding this simple rule, you can confidently navigate the world of Java arrays without encountering any unexpected exceptions. Happy coding!
What is arrayindexoutofbounds in Java?
Java lets you create and work with arrays, which are like organized lists of data. Each item in an array has a number called an index that tells you its position. The first item has an index of 0, the second has an index of 1, and so on.
If you try to access an item in the array using an index that’s outside the allowed range, Java throws an ArrayIndexOutOfBoundsException. This means you’re trying to access an item that doesn’t exist!
Think of it like trying to reach for a book on a shelf that’s beyond the edge of the shelf. You can’t get to it!
Here’s why this happens:
Negative Indices: You can’t have negative indices in an array. The smallest allowed index is always 0.
Indices Too Big: You can’t use an index that’s equal to or greater than the size of the array. For example, if your array has 5 elements, the largest allowed index is 4.
What to Do?
Double-Check Your Code: Carefully examine how you’re using array indices in your code. Ensure you’re working within the valid range.
Use `length` Property: Java provides a handy `length` property for arrays. Use it to get the size of your array and make sure your indices stay within bounds.
Handle Exceptions: You can use a `try-catch` block to catch an ArrayIndexOutOfBoundsException and handle it gracefully. This lets your program continue running even if an invalid index is used.
Let me give you a real-world example: Imagine you’re at a library, and the shelves are numbered from 1 to 10. If you ask the librarian for book number 11, they’ll tell you that book doesn’t exist on that shelf. It’s similar to how Java throws an ArrayIndexOutOfBoundsException when you try to access an item in an array using an index that’s not valid.
See more new information: linksofstrathaven.com
Java Try Catch Index Out Of Bounds: Handling Array Exceptions
Let’s dive into the world of Java exceptions, specifically the dreaded “ArrayIndexOutOfBoundsException”. We’ll explore what it is, how it happens, and how to gracefully handle it.
Understanding the Exception
Imagine you have a box of delicious cookies. You want to grab the third cookie, but you only have two in the box! That’s kind of what happens with “ArrayIndexOutOfBoundsException” in Java. It’s like trying to access a cookie that doesn’t exist.
In Java, arrays have a fixed size. When you create an array, you specify how many elements it can hold. If you try to access an element that’s beyond the array’s boundaries, the program throws an “ArrayIndexOutOfBoundsException”.
Think of it like this:
Array: Your box of cookies
Index: The position of a specific cookie (starting from 0)
ArrayIndexOutOfBoundsException: Trying to grab a cookie that isn’t in the box
Real-World Example
“`java
public class CookieExample {
public static void main(String[] args) {
String[] cookies = {“Chocolate Chip”, “Oatmeal Raisin”}; // Our box of cookies (array)
System.out.println(cookies[2]); // Trying to grab the 3rd cookie (index 2)
}
}
“`
This code will throw an “ArrayIndexOutOfBoundsException”. Why? Because we only have two cookies (index 0 and 1). The program tries to access `cookies[2]`, which doesn’t exist, causing the exception.
Why It’s Important
Let’s face it, no one wants their program crashing with a nasty error message. “ArrayIndexOutOfBoundsException” can cause your program to terminate abruptly, leading to unexpected behavior. That’s where try-catch blocks come in.
The Power of Try-Catch
Think of a try-catch block as a safety net. It lets you gracefully handle potential errors, like our cookie-grabbing problem.
Here’s the general structure:
“`java
try {
// Code that might throw an exception (like accessing an array out of bounds)
} catch (ArrayIndexOutOfBoundsException e) {
// Code to handle the exception
}
“`
Explanation:
1. try: This is where you place the code that could potentially cause an exception (like accessing an array out of bounds).
2. catch: This block catches the specific exception you’re expecting (in this case, “ArrayIndexOutOfBoundsException”).
3. e: This is a variable representing the exception object that was caught. It provides information about the error.
How to Fix the Cookie Example
Let’s fix our cookie example using a try-catch block:
“`java
public class CookieExample {
public static void main(String[] args) {
String[] cookies = {“Chocolate Chip”, “Oatmeal Raisin”};
try {
System.out.println(cookies[2]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Oops! There’s no cookie at that position!”);
}
}
}
“`
Now, when we try to access `cookies[2]`, the program won’t crash. Instead, the catch block will catch the exception, print a friendly message, and continue running.
Common Causes of ArrayIndexOutOfBoundsException
Incorrect array size: You might have created an array with a smaller size than you need, leading to an attempt to access a nonexistent element.
Off-by-one error: This is a classic mistake! You might be using an index that’s one position off the valid range. For example, if your array has 5 elements, the valid index range is 0 to 4, not 0 to 5.
Looping out of bounds: Be careful when using loops to iterate through arrays. Ensure your loop conditions prevent going beyond the array’s boundaries.
User input: If you’re getting array indices from user input, always validate the input to make sure it’s within the valid range.
Best Practices
Always use try-catch blocks: Don’t rely on hope! Use try-catch blocks to handle potential exceptions gracefully.
Catch the right exception: Catch the specific exception you’re expecting, like “ArrayIndexOutOfBoundsException”.
Don’t ignore exceptions: Even if you handle an exception, make sure you do something meaningful with it. Log the error, display a message to the user, or take appropriate action based on the situation.
Validate user input: If you’re relying on user input for array indices, always validate the input to avoid exceptions.
Debugging Tips
Read the error message: The error message usually provides helpful information about the problem. Pay attention to the index that caused the exception.
Use a debugger: Step through your code with a debugger to see exactly what’s happening at each step.
Print array indices: Add print statements to display the array index you’re accessing to help you identify the source of the problem.
Alternative Techniques
List: If you don’t know the size of your data in advance, consider using a List. Lists are dynamically resizable, so you don’t have to worry about fixed array sizes.
Array Lists: They are a great option if you need to handle variable-sized collections of data.
Maps: If you need to store key-value pairs, a Map is a suitable data structure.
FAQs
Q: Why do we need to handle ArrayIndexOutOfBoundsException?
A: Unhandled exceptions can lead to crashes and unexpected program behavior. Handling exceptions gracefully ensures that your program continues running smoothly even if errors occur.
Q: Can I use a different exception type to handle ArrayIndexOutOfBoundsException?
A: While you can catch the general `Exception` type, it’s best practice to catch specific exceptions like “ArrayIndexOutOfBoundsException” to handle them appropriately. This makes your code more readable and helps you identify the specific problem.
Q: What if I want to access an element that might not exist in the array?
A: You can use the `length` property of the array to check if the index is valid before accessing it. Here’s an example:
“`java
if (index >= 0 && index < array.length) {
// Access the element at the given index
} else {
// Handle the case where the index is invalid
}
```
Q: How can I make my code more robust when dealing with arrays?
A: Use a try-catch block, validate user input, and use techniques like List or ArrayList when necessary.
Q: Is there a way to prevent ArrayIndexOutOfBoundsException entirely?
A: While you can't always prevent it completely, you can significantly reduce the chances by implementing the best practices and techniques mentioned above.
We've covered a lot of ground, and you're now equipped to handle "ArrayIndexOutOfBoundsException" like a pro. Remember, catching exceptions makes your code more robust and reliable, preventing unexpected crashes. Happy coding!
java – try catch ArrayIndexOutOfBoundsException? – Stack Overflow
try { array[index] = someValue; } catch(ArrayIndexOutOfBoundsException exception) { handleTheExceptionSomehow(exception); } Or do as @Peerhenry suggests Stack Overflow
Array Index Out Of Bounds Exception in Java – GeeksforGeeks
2. Using Try-Catch: Consider enclosing your code inside a try-catch statement and manipulate the exception accordingly. As mentioned, Java won’t let you GeeksForGeeks
arrays – Checking out of bounds in Java – Stack Overflow
Simply use: boolean inBounds = (index >= 0) && (index < array.length); Implementing the approach with try-catch would entail catching an Stack Overflow
How to handle a java.lang.IndexOutOfBoundsException in Java?
In Java programming, IndexOutOfBoundsException is a runtime exception. It may occur when trying to access an index that is out of the bounds of an array. GeeksForGeeks
How to handle Java Array Index Out of Bounds Exception?
You can handle this exception using try catch as shown below. Example import java.util.Arrays; import java.util.Scanner; public class AIOBSampleHandled { TutorialsPoint
Java ArrayIndexOutOfBoundsException | Baeldung
ArrayIndexOutOfBoundsException occurs when we access an array, or a Collection, that is backed by an array with an invalid index. This means that the index is Baeldung
How to Handle java.lang.IndexOutOfBoundsException in Java
The java.lang.IndexOutOfBoundsException in Java is thrown when an index used in arrays, lists, or strings is not valid. A valid index must be a number from 0 up to Rollbar
How to Fix the Array Index Out Of Bounds Exception in Java
The ArrayIndexOutOfBoundsException is one of the most common errors in Java. Since a Java array has a range of [0, array length – 1], when an attempt is made to Rollbar
How to fix an Array Index Out Of Bounds Exception in
try { int x = numbers[index]; System.out.println(x); } catch (ArrayIndexOutOfBoundsException e) { System.out.println(“Error: Index is out of bounds.”); It’s also important to note that you should always check FusionReactor
java.lang.ArrayIndexOutOfBoundsExcepiton in Java with Examples
The java.lang.ArrayIndexOutOfBoundsException is one of the most common exceptions in java. It occurs when the programmer tries to access the value of geeksforgeeks.org
Java Exceptions – Learn Exceptions In Java #43
Try Catch Java Tutorial #46
Array Index Out Of Bounds Exception In Java
Learn Java Programming – Try/Catch Indexoutofboundsexception Tutorial
Out Of Bound Exception In Java
6.7 Array In Java Tutorial With Example Arrayindexoutofboundsexception
46) Array Index Out Of Bounds Exception | Java With Ali
Link to this article: java try catch index out of bounds.
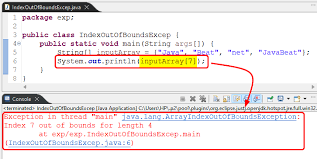
See more articles in the same category here: https://linksofstrathaven.com/how