Should arrays start at 0 or 1?
However, there’s a good reason why arrays start at 0. It’s all about how computers store and access data in memory. Think of memory as a long list of numbered boxes, each capable of holding a piece of data. When you create an array, you’re essentially reserving a certain number of these boxes for your data. The index of each element in the array corresponds to the address of the box in memory where that element is stored.
Now, let’s imagine you have an array with 5 elements. The first element is stored in the box at memory address 0, the second element is stored in the box at memory address 1, and so on. If we started indexing from 1, the first element would be at memory address 1, the second element at 2, and so on. But this would mean that the address of the first element wouldn’t be 0, which is the starting address of the memory block allocated for the array. This inconsistency can lead to potential issues when accessing elements in the array, as the computer would need to do extra calculations to find the correct address.
By starting the index at 0, the computer can directly access the memory location of each element in the array using a simple formula:
“`
Memory Address = Starting Address + Index * Size of Element
“`
This simple formula ensures efficient and accurate memory access. Starting at 0 also makes it easier for programmers to work with arrays, as they can easily calculate the position of any element in the array.
So, even though it may seem counterintuitive at first, starting arrays at 0 is a fundamental aspect of programming that helps ensure efficiency and consistency in memory management.
Does MATLAB begin counting from 0 or 1?
Imagine you have a matrix with three rows and four columns. In MATLAB, you can access the elements using row and column indices. The top-left element is accessed by specifying the first row (index 1) and the first column (index 1). The element in the second row and third column would be accessed using the indices (2, 3). This intuitive system directly reflects the way matrices are typically represented and manipulated in mathematical contexts. This straightforward approach, where indices start at 1, makes MATLAB code more readable and understandable, especially when working with mathematical formulas and concepts. You don’t need to mentally adjust indices from zero-based systems used in some programming languages.
How to make a MATLAB array start from 0?
However, you can still achieve this by using logical indexing. Think of it like using a special “filter” for your array.
Here’s how it works:
Create a logical vector with a logical 0 in the position you want to ignore. For instance, let’s say you have an array with 5 elements and you want to ignore the second element (which is at index 2). Your logical vector would look like this: `[1 0 1 1 1]`. Notice how the second element (index 2) is set to 0 (false).
Use this logical vector to index into your array. This will essentially “skip over” the element at the index where the logical vector has a 0.
Let’s look at an example:
“`matlab
% Create a sample array
myArray = [10 20 30 40 50];
% Create a logical vector to ignore the second element
myLogicalVector = [1 0 1 1 1];
% Use logical indexing to get a new array, skipping the second element
newArray = myArray(myLogicalVector);
% Display the result
disp(newArray);
“`
The output would be:
“`
10 30 40 50
“`
Important Note: This method doesn’t actually change the original array to start at index 0. It just helps you work with your data as if the element at index 2 wasn’t there.
You can also use logical indexing to manipulate multiple elements within your array. For example, to ignore the second and fourth elements, you would use the logical vector `[1 0 1 0 1]`.
Remember that logical indexing is a powerful tool for working with data in MATLAB, allowing you to selectively access and manipulate elements in your arrays.
Can I start array with 1?
Imagine an array like a street with houses numbered 0, 1, 2, and so on. When you want to find a specific house, you use its address, or index, to locate it. In programming, this index is like the address of the element you want to access in the array.
So, why start with 0? It’s more efficient for computers to work with arrays this way. Starting at 0 allows them to easily calculate the memory location of each element in the array.
Think of it like this: If you have an array with 5 elements, the computer needs to store these elements in memory. Starting at 0 means that the first element is stored at memory address 0, the second at memory address 1, and so on. This makes it quick and easy for the computer to find the element you need, without having to do any extra calculations.
While it might seem a little odd at first, understanding how arrays are indexed from 0 is essential for working with them effectively. Once you get the hang of it, it becomes a natural part of programming!
Do arrays start at 1 in MATLAB?
Think of it this way: in mathematics, we often refer to the first element of a sequence or matrix as the “first element” or “element at position 1.” MATLAB follows this same convention.
Let’s look at a simple example:
“`matlab
my_array = [1, 2, 3, 4, 5];
“`
In this example, the first element of `my_array` is 1, and you can access it using `my_array(1)`. The second element is 2, accessible as `my_array(2)`, and so on.
There are several reasons why MATLAB uses 1-based indexing:
Consistency with mathematical notation: As mentioned earlier, this makes it easier to translate mathematical equations and formulas directly into code.
Improved readability: For many users, starting array indices at 1 makes code more intuitive and easier to read.
Historical reasons: When MATLAB was first developed, 1-based indexing was a common convention in numerical computing.
While other languages might use 0-based indexing, it’s important to remember that MATLAB’s choice of 1-based indexing is a deliberate one. It makes MATLAB more consistent with mathematical conventions and can ultimately lead to more efficient and readable code.
Does array count start at 0 or 1?
Think of it this way: When you have an array with four items, it actually has five elements, but the last element is at index three. It might seem strange at first, but this system makes it easier for computers to process information in arrays. It also makes calculations for memory allocation and indexing faster. It’s like a secret code that the computer uses to keep track of things efficiently.
For example, let’s say we have an array called my_array that holds the values 10, 20, 30, and 40. We can access each value by its index:
my_array[0] would give us 10 (the first element)
my_array[1] would give us 20 (the second element)
my_array[2] would give us 30 (the third element)
my_array[3] would give us 40 (the fourth element)
So remember, when you’re working with arrays, always start counting from zero!
Is true 0 or 1 in MATLAB?
This means you can use them in logical operations like AND, OR, and NOT. For example, 1 && 0 would evaluate to 0 (false) because AND requires both sides to be true (1).
However, you can also use these logical values in mathematical calculations. For instance, 1 + 0 would evaluate to 1 because MATLAB treats 1 and 0 as numerical values in this context.
Now, you might be wondering about the boolean operator mentioned earlier. This operator is specific to Stateflow charts, a visual programming environment within MATLAB used for designing control systems. In Stateflow, the boolean operator can be used to evaluate conditions within a chart. It returns a logical 1 (true) if the condition is met and a logical 0 (false) if it’s not.
However, in the core MATLAB environment, you don’t need the boolean operator. You can directly use logical operations with numerical values, like 1 and 0, to represent true and false. For example, you could use the logical function to convert a numerical value to a logical value.
Let’s say you have a variable `x` with a value of `5`. You can use the `logical` function to check if `x` is greater than `0`:
“`matlab
x = 5;
is_positive = logical(x > 0);
“`
This would assign the value true (1) to the variable `is_positive` because `x` is indeed greater than `0`.
Essentially, MATLAB uses a consistent system for representing logical values, even though it sometimes leverages numerical values for this purpose. Understanding this system is crucial for working effectively with logical operations and conditions in your MATLAB programs.
Does MATLAB index from 0 or 1?
Why does MATLAB use 1-based indexing? It’s primarily because MATLAB was developed with engineers and scientists in mind. These users are often accustomed to working with mathematical formulas and equations, where variables and indices typically start at 1. This convention makes MATLAB code more consistent with established mathematical notations. For example, consider a vector x with elements x1, x2, x3, and so on. In MATLAB, you would access the second element using x(2), which aligns naturally with the mathematical notation x2.
In contrast, 0-based indexing, used in languages like Python and C, is more commonly associated with computer science applications. These languages often deal with memory addresses, where the first address is typically considered 0. This convention helps optimize memory management and allows for efficient addressing of data.
While MATLAB uses 1-based indexing for arrays and matrices, it actually does support 0-based indexing in certain scenarios, such as when working with structures or cell arrays. However, the default behavior for array and matrix indexing remains 1-based, reflecting its origins in the world of mathematics and engineering.
Why does MATLAB count from 1?
The concept of “1-based indexing” in MATLAB originates from the mathematical tradition of representing matrices. For instance, if you have a matrix with `m` rows and `n` columns, the elements within the matrix are indexed as follows:
| Element | Index |
|—|—|
| First element in the first row | (1,1) |
| Second element in the first row | (1,2) |
| … | … |
| First element in the second row | (2,1) |
| Second element in the second row | (2,2) |
| … | … |
| Last element in the last row | (m,n) |
This “1-based indexing” is consistent with the way matrices are typically represented in mathematical notation. It’s a convention that makes MATLAB more intuitive for users familiar with linear algebra and matrix operations. This convention also makes it easier for MATLAB to interact with other mathematical software and libraries that use the same indexing system.
So, when you work with MATLAB and see the first element of an array indexed as `A(1)`, it’s a natural consequence of MATLAB’s origins and its focus on matrix manipulation. This system ensures consistency with mathematical conventions, which contributes to MATLAB’s elegance and efficiency in handling linear algebra tasks.
See more here: Does Matlab Begin Counting From 0 Or 1? | Matlab Array Start At 0 Or 1
See more new information: linksofstrathaven.com
Matlab Array Start At 0 Or 1: The Truth Revealed
You’re probably wondering if MATLAB arrays start at 0 or 1. Well, the answer is 1. But it’s not quite as simple as that! Let me explain why and how this can sometimes be a bit confusing.
The Basics of MATLAB Arrays
MATLAB stands for “Matrix Laboratory”, and it’s all about matrices, which are essentially arrays in their purest form. In MATLAB, a matrix (and thus an array) is a collection of elements arranged in rows and columns.
Now, when we talk about indexing in MATLAB, we’re basically talking about how we refer to specific elements within the array. Let’s imagine a simple array with numbers:
“`matlab
A = [1 2 3 4 5];
“`
In this example, `A` is an array containing five numbers.
The Power of 1-Based Indexing
In MATLAB, the indexing always starts at 1. So, to access the first element in `A`, we would use `A(1)`, which gives us `1`. If we want the third element, it’s `A(3)`, which gives us `3`. It seems pretty intuitive, right?
Why Indexing Starts at 1
MATLAB’s 1-based indexing comes from its historical roots in linear algebra, a field of mathematics where matrices are heavily used. In linear algebra, the first row and column of a matrix are traditionally labeled as 1.
Now, let’s dive a bit deeper into the world of programming and see where the confusion might arise.
The 0-Based Indexing World
Many other programming languages, like C, C++, Java, and Python, use 0-based indexing. In these languages, the first element of an array is accessed using the index `0`.
Let’s say you’re used to coding in Python and suddenly switch to MATLAB. You might instinctively try to access the first element using `A(0)`. This won’t work in MATLAB. You’ll get an error, because the first element is at `A(1)`.
The Case of `linspace`
You might have come across the `linspace` function in MATLAB. This function creates a linearly spaced vector, and you can specify the number of elements you want in the vector.
For example:
“`matlab
B = linspace(0, 1, 5);
“`
This code creates a vector `B` with 5 elements, evenly spaced between 0 and 1. But here’s the catch: even though the first element in `B` is 0, the indexing still starts at 1. So, `B(1)` gives you `0`, `B(2)` gives you `0.25`, and so on.
The Bottom Line
MATLAB’s 1-based indexing might feel a little strange if you’re coming from a 0-based indexing background. But, it’s how MATLAB has always worked, and it’s deeply rooted in its linear algebra origins.
Just remember to start your indexing at 1 in MATLAB, and you’ll be good to go.
Common MATLAB Indexing Pitfalls and Best Practices
Now that we’ve covered the basics, let’s discuss some common mistakes and best practices that will help you avoid frustration when working with arrays in MATLAB.
1. Out-of-Bounds Indexing:
This is a common error, especially when you’re working with large arrays or multidimensional arrays. If you try to access an element that doesn’t exist within your array (for instance, if you try to access `A(6)` when your array `A` only has 5 elements), MATLAB will throw an error.
Best Practice: Always double-check the dimensions of your array before accessing elements, and use `size` function or `length` function to get the size of your array.
2. Negative Indexing:
Negative indexing refers to accessing elements from the end of the array. For example, `A(-1)` refers to the last element of `A`, `A(-2)` refers to the second-to-last element, and so on.
Best Practice: Use negative indexing carefully, as it can be less intuitive and might lead to confusion. If you’re accessing elements from the end of the array, consider using `end` instead. For instance, `A(end)` is a better way to access the last element of `A`.
3. Logical Indexing:
This is a powerful technique that lets you access elements based on a condition. You can use logical expressions to create a logical index that selects specific elements.
Best Practice: When dealing with logical indexing, ensure that your logical expression correctly identifies the elements you want to select. Make use of logical operators like `&` (AND), `|` (OR), and `~` (NOT) for more complex conditions.
4. Multidimensional Arrays:
Indexing multidimensional arrays can be slightly more complex. In MATLAB, you can use multiple indices to access specific elements in a multidimensional array. For example, `A(2,3)` accesses the element in the second row and third column of a 2D array `A`.
Best Practice: Always use the correct number of indices for the dimensions of your array, and ensure that the indices are within the bounds of the array.
FAQs
1. Why doesn’t MATLAB use 0-based indexing like other languages?
MATLAB’s 1-based indexing is rooted in its linear algebra origins, where matrices are conventionally indexed starting from 1.
2. Can I change MATLAB’s indexing to be 0-based?
While there’s no built-in way to change MATLAB’s indexing from 1-based to 0-based, you can use techniques like adding 1 to your index or subtracting 1 from your index within your code. However, this can make your code less readable and might lead to errors if you’re not careful.
3. What if I need to work with both 0-based and 1-based indexing in my MATLAB code?
If you’re working with data that uses both 0-based and 1-based indexing, you’ll need to adjust your indexing accordingly. This might require using conditional statements or functions to handle the different indexing schemes.
4. What are some common mistakes people make with indexing in MATLAB?
Some common mistakes include out-of-bounds indexing, negative indexing without careful consideration, and incorrect use of logical indexing.
5. How can I avoid indexing errors in my MATLAB code?
Always double-check the size and dimensions of your arrays before indexing. Use the `size` and `length` functions to get this information. Be cautious with negative indexing, and consider using `end` instead for accessing elements from the end of the array. Ensure your logical indexing expressions are correctly identifying the elements you want to access.
Remember, practicing good coding habits and understanding MATLAB’s indexing conventions will help you write more efficient and error-free MATLAB code. Happy coding!
Is there a way to start indexing with 0 in MATLAB?
rgb1(:,:,1) = 0 0.1000 0.2000 0.3000 rgb1(:,:,2) = 0 0.1000 0.2000 0.3000 rgb1(:,:,3) = 0 0.1000 0.2000 0.3000 img2 = uint8([0 1 2 3]); % class uint8 rgb2 = MathWorks
Creating an array of given size and increment – MATLAB
I simply want to create an array with a known start, increment and number of elements. Obviously if I have start, increment, end I can use the colon operator and if I MathWorks
How to get indexing to start at 0? – MATLAB Answers – MathWorks
In order to get MATLAB to index starting from 0, you need to create an extensive set of Object Oriented Programming classes that behave like normal numeric MathWorks
Programming with MATLAB: Arrays
For example, if we type 1:3:15 it generates an array starting with 1, then 1+3, then 1+2*3, and so on, until it reaches 15 (or as close as it can get to 15 without uomresearchit.github.io
Introduction To Matlab Array: A Complete Guide – The
Do MATLAB Arrays start at 0 or 1? MATLAB Arrays start at 1. Unlike some programming languages that use 0-based indexing, MATLAB’s indexing convention The Knowledge Academy
Is it possible to put array index as zero in matlab?
Indexing does indeed start from 1, but you can modify the indexing in your logic to map the required 0th index to the actual 1st index in the matrix/array. MathWorks
An Introduction to Matlab Arrays | Simplilearn
Do MATLAB arrays start at 0 or 1? If you’re a programmer, you’ve probably heard this before: in most programming languages, the first element of an array simplilearn.com
arrays – Why do we count starting from zero? – Computer Science …
With zero-based indexing, the expression a[i] compiles to an operation on memory address a + c*i where c is a constant representing the size of a single array stackexchange.com
Why The Array Index Start From 0
Why Array Starts At 0 || Explained In Detail
Indexing Columns And Rows | Managing Code In Matlab
Matlab 👩💻 Array And Matrix
Matlab Arrays 01 – Creating A 1D Array
Array Operations In Matlab
Matlab Zeros, Ones, And Eye Commands
Link to this article: matlab array start at 0 or 1.
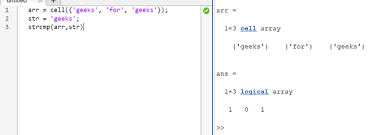
See more articles in the same category here: https://linksofstrathaven.com/how